Follow the Getting Started guide to create a new web site and load the diagram control, setting it up in Canvas mode.
1. In the page code-behind define a node template string that lists layout containers and graphics primitives from which nodes should be composed.
C#
Copy Code
|
---|
string template = @"{ component: ""GridPanel"", rowDefinitions: [""*""], columnDefinitions: [""22"", ""*""], children: [ { component: ""Rect"", name: ""Background"", pen: ""black"", brush: ""white"", columnSpan: 2 }, { component: ""Image"", name: ""Image"", autoProperty: true, location: ""Images/icon4.png"", margin: ""1"", imageAlign: ""Fit"" }, { component: ""StackPanel"", orientation: ""Vertical"", gridColumn: 1, margin: ""1"", verticalAlignment: ""Near"", children: [ { component: ""Text"", name: ""Title"", autoProperty: true, text: ""title"", font: ""Arial bold"" }, { component: ""Text"", name: ""FullName"", autoProperty: true, text: ""full name"", pen: ""blue"", padding: ""1,0,1,0"" }, { component: ""Text"", name: ""Details"", autoProperty: true, text: """", font: ""Arial 3"" } ] } ] } "; |
2. Add a new OrgChartNode class to the project and implement it as below. Its instances will represent the custom nodes posted back fro client side. The AutoJson attribute generates automatic JSON serialization .NET code to transfer values of properties defined in the template.
C#
Copy Code
|
---|
using MindFusion.Diagramming; using MindFusion.Diagramming.WebForms;
public class OrgChartNode : CompositeNode { public OrgChartNode() { }
public OrgChartNode(Diagram diagram) : base(diagram) { }
[AutoJson] public string Image { get; set; }
[AutoJson] public string Title { get; set; }
[AutoJson] public string FullName { get; set; }
[AutoJson] public string Details { get; set; } } |
3. Call RegisterItemType to register the custom class with diagram controls that will display its instances.
C#
Copy Code
|
---|
protected void Page_Init(object sender, EventArgs e) { // register custom item type diagramView.RegisterItemType( typeof(OrgChartNode), "OrgChartNode", "OrgChartNode", 100, template); nodeListView.RegisterItemType( typeof(OrgChartNode), "OrgChartNode", "OrgChartNode", 100, template); } |
4. Set up the controls and create a few nodes is Page_Load handler:
C#
Copy Code
|
---|
protected void Page_Load(object sender, EventArgs e) { // let users draw OrgChartNode objects in Canvas mode diagramView.Behavior = Behavior.Custom; diagramView.CustomNodeType = typeof(OrgChartNode); diagramView.Diagram.UndoManager.UndoEnabled = true;
nodeListView.NodeSize = nodeListView.DefaultNodeSize = new SizeF(60, 25);
if (!IsPostBack) { var diagram = diagramView.Diagram;
var node1 = new OrgChartNode(); node1.Bounds = new RectangleF(25, 15, 60, 25); node1.Title = "CEO"; node1.FullName = "John Smith"; node1.Details = "Our beloved leader.\r\n" + "The CEO of this great corporation."; node1.Image = "Images/ceo.png"; diagram.Nodes.Add(node1); nodeListView.AddNode(node1);
var node2 = new OrgChartNode(); node2.Bounds = new RectangleF(25, 55, 60, 25); node2.Title = "CTO"; node2.FullName = "Bob Smith"; node2.Details = "The technology chief of this great corporation."; node2.Image = "Images/cto.png"; diagram.Nodes.Add(node2); nodeListView.AddNode(node2);
var node3 = new OrgChartNode(); node3.Bounds = new RectangleF(95, 55, 60, 25); node3.Title = "HR"; node3.FullName = "Mary Johnson"; node3.Details = "Human resources and staff development."; node3.Image = "Images/hr.png"; diagram.Nodes.Add(node3); nodeListView.AddNode(node3);
var node4 = new OrgChartNode(); node4.Bounds = new RectangleF(175, 55, 60, 25); node4.Title = "PR"; node4.FullName = "Diana Brandson"; node4.Details = "Public relations and media."; node4.Image = "Images/pr.png"; diagram.Nodes.Add(node4); nodeListView.AddNode(node4);
diagram.Factory.CreateDiagramLink(node1, node2); diagram.Factory.CreateDiagramLink(node1, node3); diagram.Factory.CreateDiagramLink(node3, node4); } } |
5. If you run the tutorial now, you should see this in your web browser:
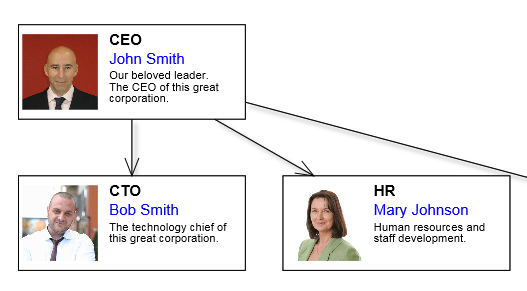