In this post we discuss how to create a drill down chart with the MindFusion.Charting for WPF tool. Our main chart will be a pie chart, where each peace shows some aggregate data. When clicked, a new chart pops up – a bar chart, which shows details about the clicked piece.
The Data
For the data we use an ObservableCollection called CompanyExpenses. It contains objects of type Expenses. The Expenses class implements INotifyPropertyChanged. Here is a code snippet:
public class Expenses : INotifyPropertyChanged
{
public Expenses(string corporationName, double marketing, double salaries,
double rawMaterials, double logistics, double administration, double production)
{
this.corporationName = corporationName;
this.marketing = marketing;
this.salaries = salaries;
this.rawMaterials = rawMaterials;
this.logistics = logistics;
this.administration = administration;
this.production = production;
}
.............
}
We have properties for the various company expenses and a property for the name of the corporation. We have a special Sum property, which gives us the total of all expenses for the corporation. This property will be used by the main chart – the pie chart:
public double Sum
{
get { return sum; }
set
{
sum = value;
OnPropertyChanged("Sum");
}
}
The Pie Chart
The pie chart displays the expenses of all 5 corporations – together with their name and their share. We use data binding, the ComapnyExpenses list provides the DataSource:
CompanyExpenses data = new CompanyExpenses();
pieChart1.DataSource = data;
In order to show the name of the company as an outer label, we must set the OuterLabelType to CustomText and bind Expenses.CorporationName to the OuterLabelPath property. We do this in XAML:
my:PieSeries OuterLabelOffset="30" OuterLabelPath="CorporationName" OuterLabelType="CustomText" DataPath="Sum" InnerLabelType="Percents" Name="pieSeries1" DetachedPiecesList="20"
The Sum property, which we mentioned above, provides data for the chart. The brushes are set with the brush editor in the property grid.
Hit Testing
We use the charting component’s HitTest method to detect when a piece was clicked and to show a bar chart with the respective data. PiePiece.PieceIndex gives us the index of the clicked piece. We use the Control.MouseDown event to detect mouse clicks.
private void pieChart1_MouseDown(object sender, MouseButtonEventArgs e)
{
List result =
pieChart1.HitTest(e.GetPosition(pieChart1));
if (result.Count > 0 && result[0] is MindFusion.Charting.Wpf.PiePiece)
{
MindFusion.Charting.Wpf.PiePiece piece =
result[0] as MindFusion.Charting.Wpf.PiePiece;
Details d = new Details(data[piece.PieceIndex]);
d.Show();
}
}
The HitTest method returns a collection of ChartElement objects. In our case we don’t have several ChartElements that overlap each other and might be clicked simultaneously, that’s why we take the first ChartElement.
The Detailed Chart
The detailed chart is a bar chart that displays the data for a single Expenses object. We set the labels at the X-axis to display the type of the expense:
barChart1.XAxisSettings.LabelType = MindFusion.Charting.Wpf.LabelType.CustomText;
barChart1.XLabels = new List() { "Marketing", "Salaries", "Raw Materials", "Logistics", "Administration", "Production"};
barChart1.XAxisSettings.LabelRotationAngle = 30;
barChart1.XAxisSettings.CustomLabelPosition = MindFusion.Charting.Wpf.CustomLabelPosition.ChartDataPoints;
When we create the Details window, we pass as argument the Expenses object the chart refers to:
public Details( Expenses expenses)
{
barSeries1.YData = expenses.ExpensesList;
…
}
The data for the bar chart comes from the list of the expenses, which is a DoubleCollection.
Here is a screenshot of the final drill down chart:
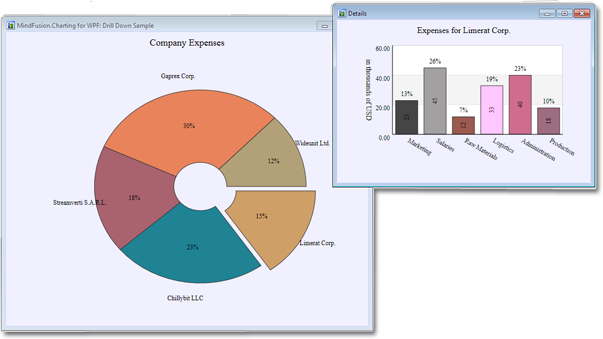
The main pie chart with the bar chart that shows details for the clicked pie piece.
You can download the complete source code for the project from this link:
Download MindFusion.Charting Drill Down Sample