In this blog post we will create the following timetable that shows the registered ski classes for a ski school week per week:
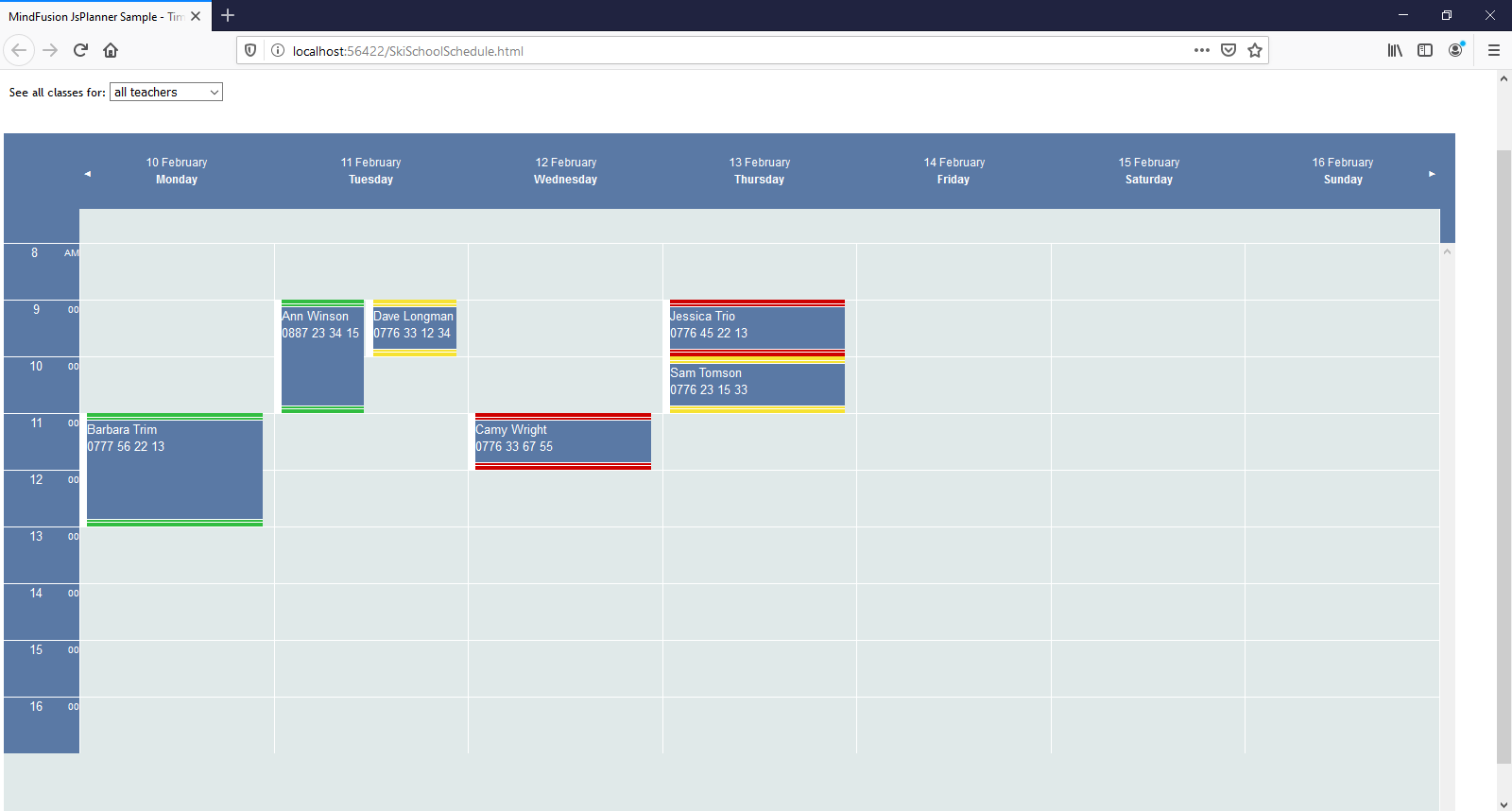
We use MindFusion JavaScript Scheduler to create the timetable. We use the BaseForm class to create the custom form that allows us to choose the level of the skier.
This is not possible in the standard appointment form that comes with the scheduling library.
I. General Settings
We need to add a DIV element with an id at the location where we want the timetable to appear on the page. Note that the size of the DIV determines the size of the calendar:
MindFusion.Scheduling requires a reference to MindFusion.Scheduling:
<script src="MindFusion.Scheduling.js" type="text/javascript"></script>
<script src="SkiSchoolSchedule.js" type="text/javascript"></script>
<script src="SkiStudentForm.js" type="text/javascript"></script>
We also add a reference to two JavaScript files that will contain the source code for our application: SkiSchoolSchedule and SkiStudentForm. Finally we add a reference to the CSS file that defines the theme used by the timetable – business.css:
<link rel="stylesheet" type="text/css" href="themes/business.css">
The Scheduling library offers a variety of CSS themes, which you can customize or use as a template to create new ones.
II. The Timetable
In the code-behind file we add a mapping to MindFusion.Scheduling namespace and then create the Calendar instance using the id of the DIV element that will render it:
var p = MindFusion.Scheduling;
// create a new instance of the calendar
calendar = new p.Calendar(document.getElementById("calendar"));
// set the view to Timetable, which displays the allotment of resources to distinct hours of a day
calendar.currentView = p.CalendarView.Timetable;
//set the theme to business as referenced
calendar.theme = "business";
We also set the currentView property to CalendarView and specify the theme – business as the name of the CSS file that we referenced.
The timetableSettings class exposes a dates property where we add the initial dates that will appear in the view:
//get the current date
var currDay = p.DateTime.today();
calendar.timetableSettings.dates.clear();
/* add dates to the timetable in such manner that always a full week
rom Mo to Su will be visible */
for(var i = 1; i < 8; i++)
{
calendar.timetableSettings.dates.add(currDay.addDays(-1 * currDay.dayOfWeek + i));
}
We get the current date and add in a cycle all 7 days of the week that contains it. We set the scrollStep property to 7, which indicates the number of days that will appear by initial click on one of the navigation arrows in the header:
// set the number of days to scroll with when a navigation button is clicked
calendar.timetableSettings.scrollStep = 7;
We will also use the startTime and endTime properties to indicate the start and and time of the timetable for each day. Those properties show the time as interval added to the start of the current day, in minutes. Thus a startTime value of 300 means the timetable starts 5 hours (5*60 min.) after midnight of the respective day:
// set the start time to 8:00 AM
calendar.timetableSettings.startTime = 480;
// set the end time to 18:00 PM
calendar.timetableSettings.endTime = 1020;
III. The Custom Form
We don’t want to use the standard form for creating appointments. We will create a custom one, you can see the difference between them at this image:
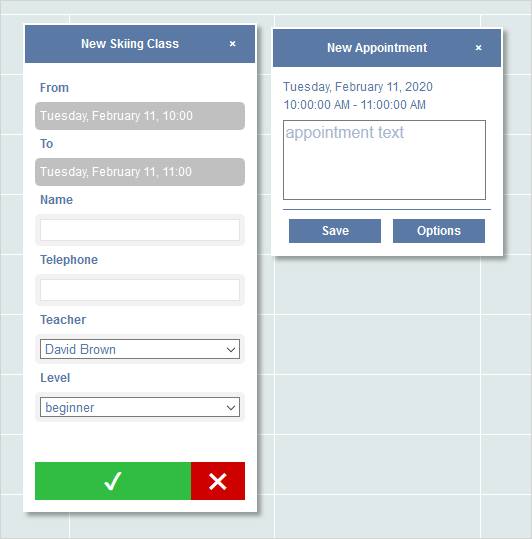
The left one is the custom form while to the right you can see the standard New Appointment form for calendar events.
The custom form for creating and editing ski lessons derives from the BaseForm class. Here is its constructor:
var SkiStudentForm = function (calendar, item, type)
{
p.BaseForm.call(this, calendar, item);
this._id = "SkiStudentForm";
this._type = type;
if(type == "new")
this.headerText = "New Skiing Class";
else
this.headerText = "Edit Skiing Class";
this.levels = [
{ value: 0, text: "beginner" },
{ value: 1, text: "intermediate" },
{ value: 2, text: "advanced" }
];
}
We want each form to have a reference to the Calendar, to the Item that was created and a type. The type is simply a string and we recognize two types: new and edit e.g. whether we create a new class or edit an existing one.
Note that in the constructor we initialize a new variable called levels, which will provide data for the combo box with options for the skiing level of the student.
Then we call the prototype methods of the class and the constructor so we can initialize instances of SkiStudentForm:
SkiStudentForm.prototype = Object.create(p.BaseForm.prototype);
SkiStudentForm.prototype.constructor = SkiStudentForm;
The two methods that are responsible for drawing the contents of a custom BaseForm and its buttons are drawContent and drawButtons
We start with the drawContent method, where we create the first row:
SkiStudentForm.prototype.drawContent = function ()
{
p.BaseForm.prototype.drawContent.call(this);
var content = this.content;
var row = this.row();
row.className = "header-row";
row.innerText = "From";
content.appendChild(row);
row = this.row();
row.className = "data-row";
row.innerHTML = this.item.startTime.toString("dddd, MMMM d, HH:00", this.formatInfo);
content.appendChild(row);
........................
}
We call the prototype of the drawContent method and we add a new row element. The row is an empty DIV. Then we add another row, this one contains a string. The string represents the start time of the Item .
We create the combo box using the createDropDownList method. Before we create the combo box we add a new DIV with the label. Then we add another DIV and the dropDownList element to it:
// create a drop-down list for status
row = this.row();
row.className = "header-row";
row.innerHTML = "Level";
content.appendChild(row);
var control = this.createDropDownList({ id: "level", items: this.levels, initValue: this.item.tag, addEmptyValue: false });
control.element.style.width = "200px";
this.addControl(control);
row = this.row();
row.className = "input-row";
row.appendChild(control.element);
content.appendChild(row);
The drawButtons method that we implement overrides the buttons of the BaseForm with new ones, styled as we want. Here the code for the Save button:
// override BaseForm's drawButtons method to create form buttons
SkiStudentForm.prototype.drawButtons = function ()
{
var thisObj = this;
var btnSave = this.createButton(
{
id: "btnSave",
text: "✔",
events: {
"click": function click(e)
{
return thisObj.onSaveButtonClick(e);
}
}
});
btnSave.element.className = "form-button-save";
We use the createButton method to create the button, give it an id and assign a text to it. We also indicate the the click event will be handled by an implementation of the default onSaveButtonClick event for BaseForm
The Cancel button is the same, just the CSS styling for it is different. Both buttons use custom CSS class, which are assigned to them through the className property of HTML Dom elements. Here is the code for the appearance of the two buttons:
.form-buttons
{
color: #fff;
font-size: x-large;
text-align: center;
margin-top: 20px !important;
}
.form-button-save
{
width: 80%;
padding: 10px;
background-color: #31bd41 !important;
}
.form-button-cancel
{
width: 20%;
padding: 10px;
background-color: #ce0000 !important;
}
We create and render an instance of the form with the following lines of code:
var form = new SkiStudentForm(sender, item, "new");
form.showForm();
Here we create the form from an event handler for the Calendar class – we will look at that in the next section.
IV. Events
We show the student appointment form when a selection of cells is make. In order to do this we handle the selectionEnd method of the Calendar class:
// handle the selectionEnd event to show the custom form for item creation
calendar.selectionEnd.addEventListener(handleSelectionEnd);
function handleSelectionEnd(sender, args)
{
// we create a new item with the selected start and end time
var item = new p.Item();
item.startTime = args.startTime;
item.endTime = args.endTime;
item.tag = 0;
// create and show the custom form
var form = new SkiStudentForm(sender, item, "new");
form.showForm();
}
We create a new Item and we set its startTime and endTime to the start and end of the selected cell range. Then we use the current Calendar, which is provided as a sender, the newly created Item and “new” as type to create an instance of the SkiStudentForm and render it.
What shall we do if we want to use the custom form to edin an existing appointment? We will handle the itemDoubleClick event of the Calendar and show the SkiStudentForm. In this case we will get the Item that we want to edit and provide it as a parameter to the SkiStudentForm instance. We also change the type to be “edit”:
function handleItemDoubleClick(sender, args)
{
// show the custom form with data from the clicked item
var form = new SkiStudentForm(sender, args.item, "edit");
form.showForm();
}
These were the most important parts of the ski school scheduler application. The application has a few more, which we did not mention here but you can check them in code. The complete code, together with the themes and libraries used is available at:
Download the Ski School Scheduler Application
You can post technical questions, comments and recommendations about MindFusion Scheduling for JavaScript at the library online forum.
About Scheduling for JavaScript: MindFusion Js Scheduler is the right solution for all applications that need to render interactive timetables, rich event calendars, lists with appointments or resources. Fully responsive, highly customizable and easy to integrate, you can quickly program the JavaScript scheduling library according to your needs. The library supports a variety of export options, styling through themes, 6 calendar views and much more. Find out more at https://mindfusion.eu/javascript-scheduler.html